For retail and e-commerce companies, leveraging customer data effectively is crucial to improving satisfaction, enhancing retention, and increasing sales. However, advanced analyses like sentiment detection, sales trend monitoring, and churn prediction often require tools beyond Excel, forcing teams to toggle between platforms and rely on additional software. With Python now integrated into Excel, these sophisticated techniques are available directly within your spreadsheets, combining Excel’s familiarity with Python’s analytical power.
In this post, we’ll explore how Python in Excel can transform customer insights through real-world applications in sentiment analysis, sales trend analysis, and churn prediction. From identifying how customers feel about your products to predicting which subscribers might leave, each example demonstrates how Python’s capabilities can seamlessly enhance Excel’s functionality. Now, you can conduct complex analyses with ease, making data-driven decisions faster and more accessible than ever.
To start using Python in Excel, simply type “=py(“, and an editor will open, allowing you to apply Python functions, access powerful libraries, and perform advanced analysis—all without leaving your spreadsheet. Whether analyzing customer reviews or predicting churn, Python in Excel brings data science within reach for teams of any skill level.
Sentiment Analysis on Customer Reviews with Python in Excel
Every day, customers leave reviews that offer valuable insights into their experiences, satisfaction levels, and even product improvements. However, manually analyzing large volumes of reviews can be time-consuming and subjective. Python in Excel provides an efficient solution by enabling sentiment analysis directly within your spreadsheets, allowing you to categorize reviews as positive, negative, or neutral with ease.
In this example, we’ll walk through how to perform sentiment analysis on customer review data using NLTK’s tools for text processing. This approach leverages custom positive and negative word lists to classify sentiment accurately, enabling you to uncover actionable insights without complex setup.
To begin, we need to load our review data into Excel and clean it for analysis. Let’s assume we have a dataset of customer reviews, including text and additional metadata like rating scores. Once imported, Python’s Pandas library can help us format and clean the data to ensure it’s ready for sentiment analysis.
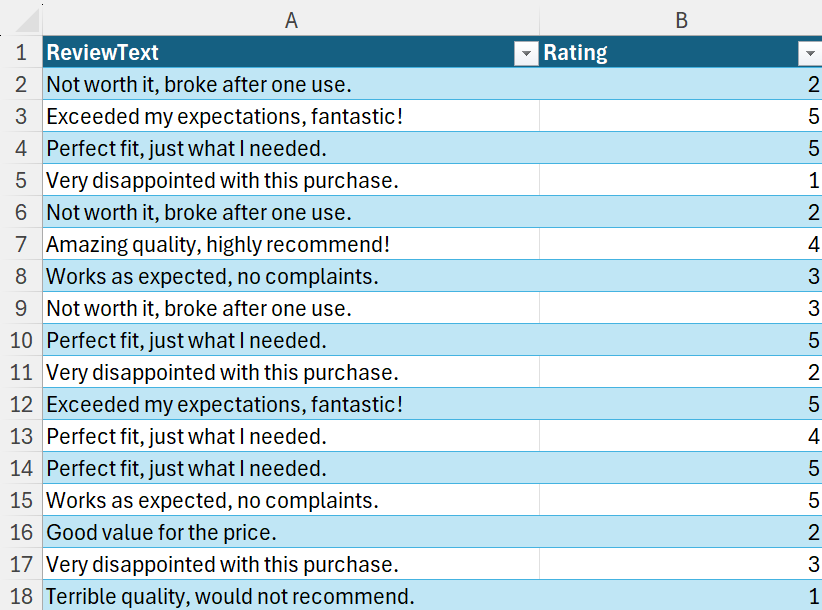
# Load review data from Excel
df = xl("Table3[#All]", headers=True)
# Import NLTK resources
import nltk
from nltk.corpus import stopwords
from nltk.tokenize import word_tokenize
# Define stopwords for cleaning
stop_words = set(stopwords.words('english'))
# Function to clean and tokenize text
def clean_text(text):
tokens = word_tokenize(text.lower()) # Lowercase and tokenize
return [word for word in tokens if word.isalpha() and word not in stop_words]
# Apply text cleaning
df['CleanedReview'] = df['ReviewText'].apply(clean_text)
This quick setup gives us an organized view of the review text and any associated ratings, providing the foundational data we’ll analyze for sentiment.
Without relying on additional libraries, we’ll use custom lists of positive and negative words to classify sentiment. This method assigns each review a sentiment based on the presence of words that indicate positive or negative emotions.
# Define positive and negative word lists
positive_words = ["good", "great", "excellent", "love", "amazing", "satisfied", "happy"]
negative_words = ["bad", "terrible", "poor", "hate", "disappointed", "unsatisfied", "worst"]
# Function to calculate sentiment based on word lists
def analyze_sentiment(review_tokens):
pos_count = sum(1 for word in review_tokens if word in positive_words)
neg_count = sum(1 for word in review_tokens if word in negative_words)
return "Positive" if pos_count > neg_count else "Negative" if neg_count > pos_count else "Neutral"
# Apply sentiment analysis
df['Sentiment'] = df['CleanedReview'].apply(analyze_sentiment)
This analysis provides each review with a sentiment score that indicates its polarity. With a quick filter, you can segment reviews by sentiment, helping you identify the overall tone of customer feedback.
With sentiment scores calculated, we can visualize the distribution of positive, neutral, and negative reviews. This overview allows us to quickly assess the general mood among customers.
# Reorder the data with specific order and colorssentiment_order = ['Negative', 'Neutral', 'Positive']colors = ['red', 'grey', 'green']
# Plot with ordered categoriesdf['Sentiment'].value_counts().reindex(sentiment_order).plot( kind='bar', color=colors)
plt.title("Sentiment Analysis of Customer Reviews")plt.xlabel("Sentiment")plt.ylabel("Number of Reviews")sns.despine()plt.show()
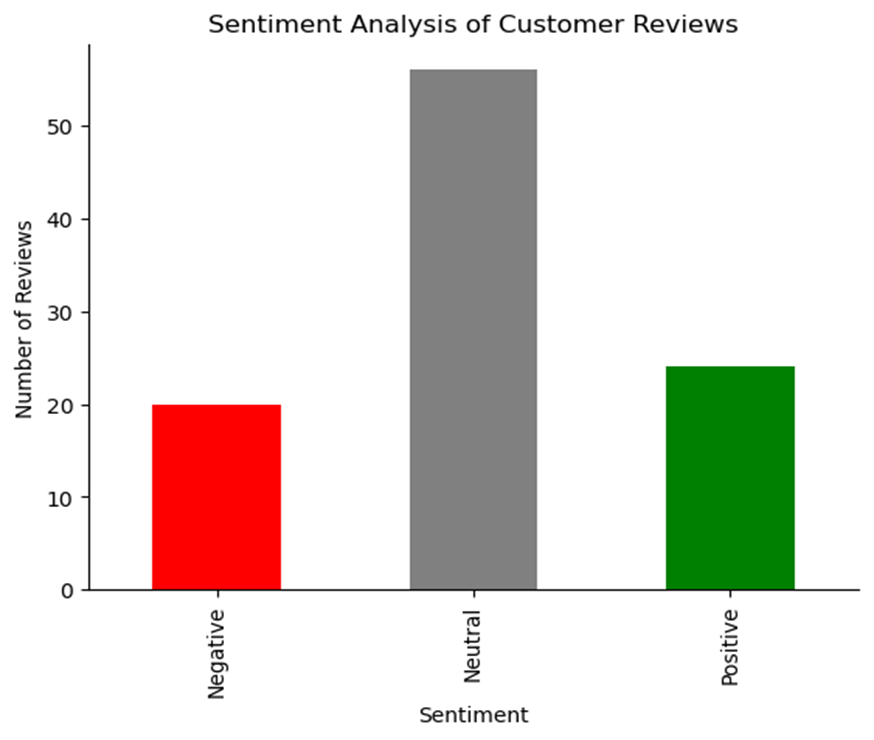
By integrating sentiment analysis into Excel using Python, retail and e-commerce teams can quickly capture customer sentiment and use that information to inform product improvements, customer support, and marketing strategies. With Python’s text analysis capabilities, you can categorize hundreds or thousands of reviews in a fraction of the time it would take manually, giving you timely insights into customer satisfaction. This seamless workflow brings powerful text analytics directly into Excel, making it an accessible, invaluable tool for customer insights.
Sales Trend Analysis by Product Category with Python in Excel
For retail and e-commerce companies, understanding sales trends by product category is essential for strategic planning and inventory management. By analyzing these trends, businesses can identify popular product lines, anticipate seasonal demand, and adjust stock levels accordingly. However, performing detailed trend analysis often requires switching between tools, especially for advanced data manipulation. With Python in Excel, you can conduct comprehensive sales trend analysis directly within your spreadsheets, making it easier to visualize and act on key insights.
In this example, we’ll use Python in Excel to analyze monthly sales trends by product category, helping identify which categories are growing, stable, or declining. This approach not only highlights trends but also enables businesses to make data-driven decisions on product placement, promotions, and stocking.
Step 1: Load and Prepare the Sales Data
Start by loading sales data into Excel and using Python to organize it by month and category. Let’s assume we have a dataset that includes product categories, sales amounts, and dates. With Python’s Pandas library, we can quickly set up this data for time series analysis.
# Load sales data from Excel tabledf = xl("SalesData[#All]", headers=True)
# Group by month and category and sum the salesdf['Month'] = df['Date'].dt.to_period('M')monthly_sales = df.groupby(['Month', 'Category'])['Sales'].sum().reset_index()monthly_sales.Month = monthly_sales.Month.dt.to_timestamp()monthly_sales
We can set the output of this Python cell to ‘Excel values’ so that the DataFrame monthly_sales spills to the grid from cell E1.
Now we can use Anaconda Toolbox to quickly create a chart visualizing the sales trends.
We create a new chart, select Line Chart then configure the Setup like this:
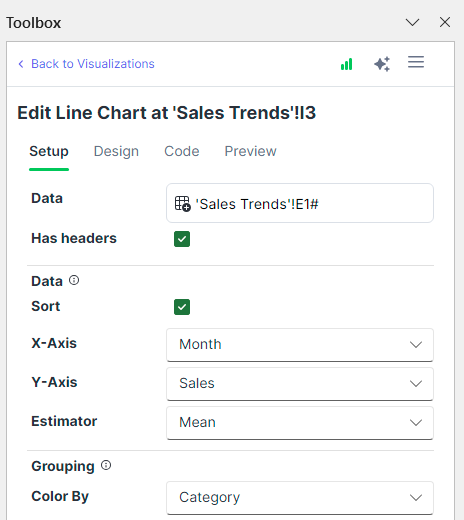
We can set some visual preferences such as color palette, chart title and line width on the Design tab.
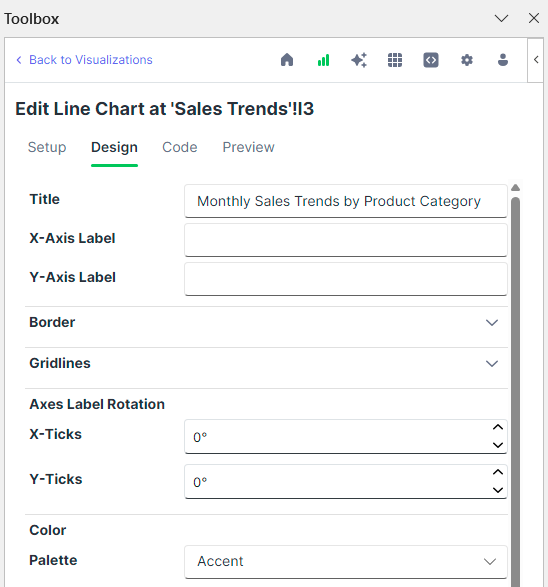
When we’re happy with our selections, we can preview the chart on the Preview tab.
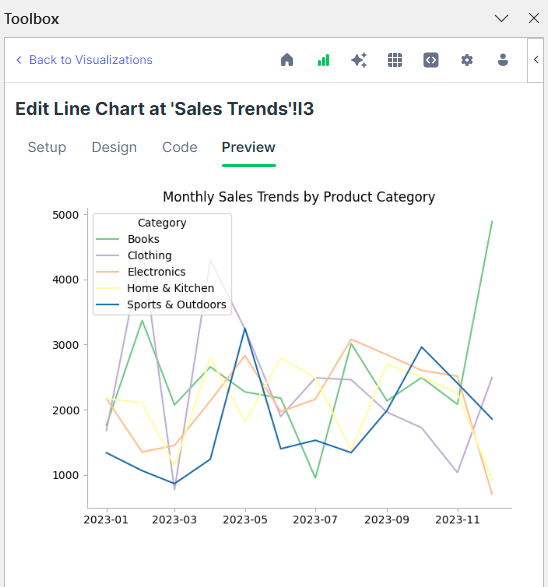
To fine-tune the chart’s appearance, we can optionally edit the generated code on the ‘Code’ tab.
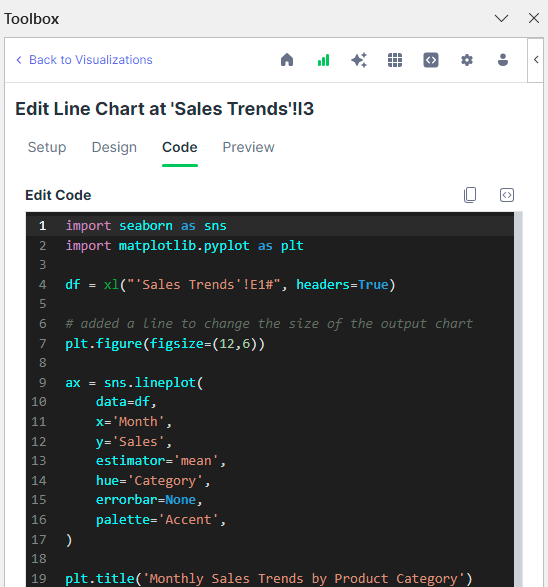
When we’ve finished with our chart setup, we can hit the ‘Create’ button to load the chart to a cell in the workbook.
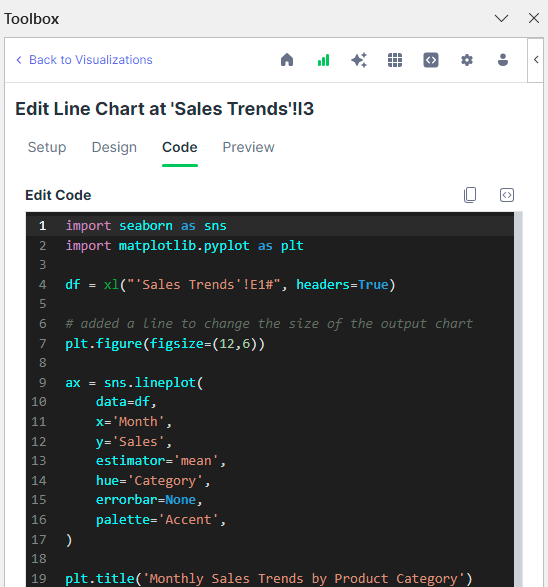
When we’ve finished with our chart setup, we can hit the ‘Create’ button to load the chart to a cell in the workbook.
The finished chart looks like this:
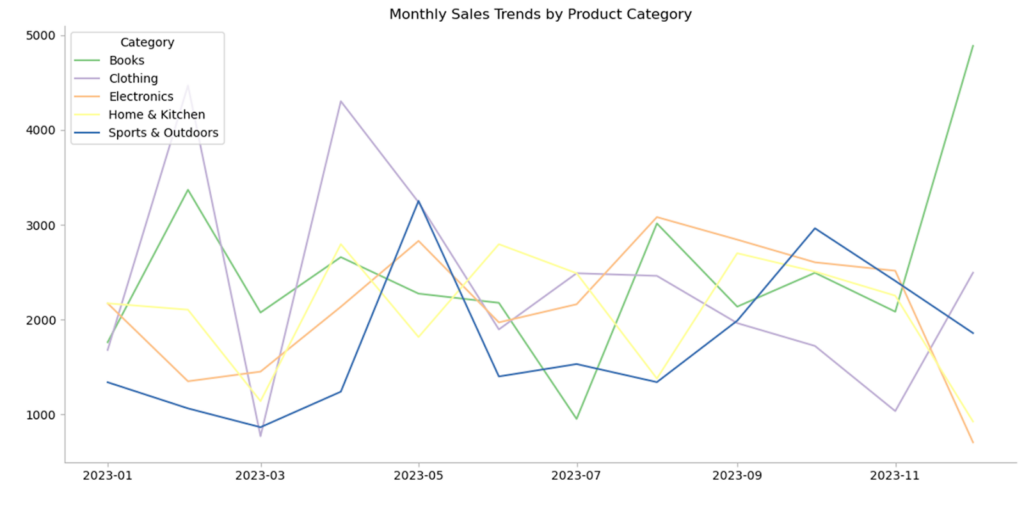
# Plot monthly sales trends by category
plt.figure(figsize=(10, 6))
for category in monthly_sales['Category'].unique():
category_data = monthly_sales[monthly_sales['Category'] == category]
plt.plot(category_data['Month'].astype(str), category_data['Sales'], label=category)
plt.title("Monthly Sales Trends by Product Category")
plt.xlabel("Month")
plt.ylabel("Sales")
plt.legend(title="Category")
plt.xticks(rotation=45)
plt.show()
# Calculate total sales and growth rate for each category
category_summary = monthly_sales.groupby('Category')['Sales'].agg(['sum', 'mean'])
category_summary['Growth Rate'] = monthly_sales.groupby('Category')['Sales'].pct_change().fillna(0).mean()
# Sort categories by total sales to identify top-performing product lines
top_categories = category_summary.sort_values(by='sum', ascending=False)
top_categories
sum | mean | Growth Rate | |
Category | |||
Books | 29880.73 | 2490.061 | 0.187185 |
Clothing | 28519.73 | 2376.644 | 0.187185 |
Electronics | 25819.73 | 2151.644 | 0.187185 |
Home & Kitchen | 25075.16 | 2089.597 | 0.187185 |
Sports & Outdoors | 21248.69 | 1770.724 | 0.187185 |
With Python in Excel, conducting a sales trend analysis by product category becomes a streamlined process. You can easily explore which product categories drive sales growth, visualize monthly trends, and calculate metrics like growth rates or total sales—all within your Excel workflow.
By combining Python’s data analysis capabilities with Excel’s familiarity, retail and e-commerce businesses can gain timely insights, adapt to seasonal demand shifts, and optimize inventory levels without needing additional tools. This seamless integration enables both analysts and non-technical team members to leverage advanced trend analysis, helping drive smarter decisions that enhance overall business performance.
Churn Prediction for E-commerce Subscribers with Python in Excel
For e-commerce businesses, customer churn, which is the rate at which customers stop engaging or cancel subscriptions, can be a significant challenge. Understanding why subscribers churn and predicting who might leave is crucial for developing effective retention strategies. Traditional tools can handle some analysis, but predicting churn requires more advanced techniques. With Python in Excel, you can now build a predictive model to identify high-risk customers directly within your spreadsheets, helping you take proactive steps to retain them.
In this example, we’ll use Python to predict churn for e-commerce subscribers based on simulated customer data. By combining data on past behavior, demographics, and purchase history, we can develop a model that flags at-risk subscribers. This approach enables businesses to target retention efforts precisely where they’re needed most.
First, load subscriber data, including relevant features like demographics, purchase frequency, and engagement scores. This data will serve as the input for our churn prediction model.
# Load subscriber data from Excel
df = xl("SubscribersData[#All]", headers=True)
The data should include features like age, engagement score, average purchase value, and a binary “Churn” column indicating whether each subscriber has churned. This data forms the basis for our predictive model.
Using Python’s scikit-learn library, we can develop a basic logistic regression model, a popular choice for binary classification problems like churn prediction. Logistic regression provides clear interpretability, making it easier to understand the impact of each feature on churn probability.
from sklearn.model_selection import train_test_splitfrom sklearn.linear_model import LogisticRegressionfrom sklearn.metrics import accuracy_score, classification_reportfrom sklearn.preprocessing import StandardScaler
# Separate features and targetX = df[['Age', 'EngagementScore', 'AvgPurchaseValue', 'NumPurchases']] # Feature columnsy = df['Churn'] # Target column
# Split the data into training and testing setsX_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
# Initialize the scaler and fit it to the training datascaler = StandardScaler()X_train = scaler.fit_transform(X_train)X_test = scaler.transform(X_test)
model = LogisticRegression()model.fit(X_train, y_train)
y_pred = model.predict(X_test)
# Evaluate model performanceprint("Accuracy:", accuracy_score(y_test, y_pred))print(classification_report(y_test, y_pred))
# Predict churn probabilities for all subscribers
df['ChurnProbability'] = model.predict_proba(X)[:, 1]
# Identify subscribers with a high churn probability
at_risk_subscribers = df[df['ChurnProbability'] > 0.5] # Threshold for high risk
at_risk_subscribers.head()
Customer | Age | EngagementScore | AvgPurchaseValue | NumPurchases | Churn | ChurnProbability |
0 | 56 | 0.46 | 271.71 | 45 | 1 | 0.930341 |
1 | 46 | 0.55 | 231.57 | 18 | 0 | 0.999997 |
3 | 60 | 0.39 | 288.63 | 21 | 0 | 1 |
4 | 25 | 0.96 | 94.52 | 15 | 0 | 0.662107 |
6 | 56 | 0.2 | 433.66 | 35 | 1 | 1 |
The ChurnProbability column provides a score between 0 and 1, representing each subscriber’s risk of churn. Subscribers with a probability above 0.5 are flagged as high-risk.
With Python in Excel, e-commerce companies can streamline churn prediction without leaving their spreadsheet environment. By using logistic regression, you can build a simple yet effective model that flags at-risk subscribers, enabling targeted interventions that improve retention. This approach saves time and empowers non-technical teams to incorporate advanced analytics directly in Excel.
Conclusion
With Python integrated into Excel, retail and e-commerce companies can take their data analysis to the next level without leaving the familiar Excel environment. From sentiment analysis that provides quick insights into customer feedback, to trend analysis that keeps inventory optimized, and churn prediction models that identify high-risk subscribers, Python in Excel offers a comprehensive toolkit for impactful decision-making.
This integration removes the need for external software and provides non-technical teams with the power to leverage advanced analytics easily. By combining the strengths of both tools, retail and e-commerce businesses can transform raw data into actionable insights that support everything from marketing and customer service to inventory and retention strategies. Whether you’re new to Python or a seasoned analyst, Python in Excel empowers you to harness data for competitive advantage, all within the software you know and use every day.